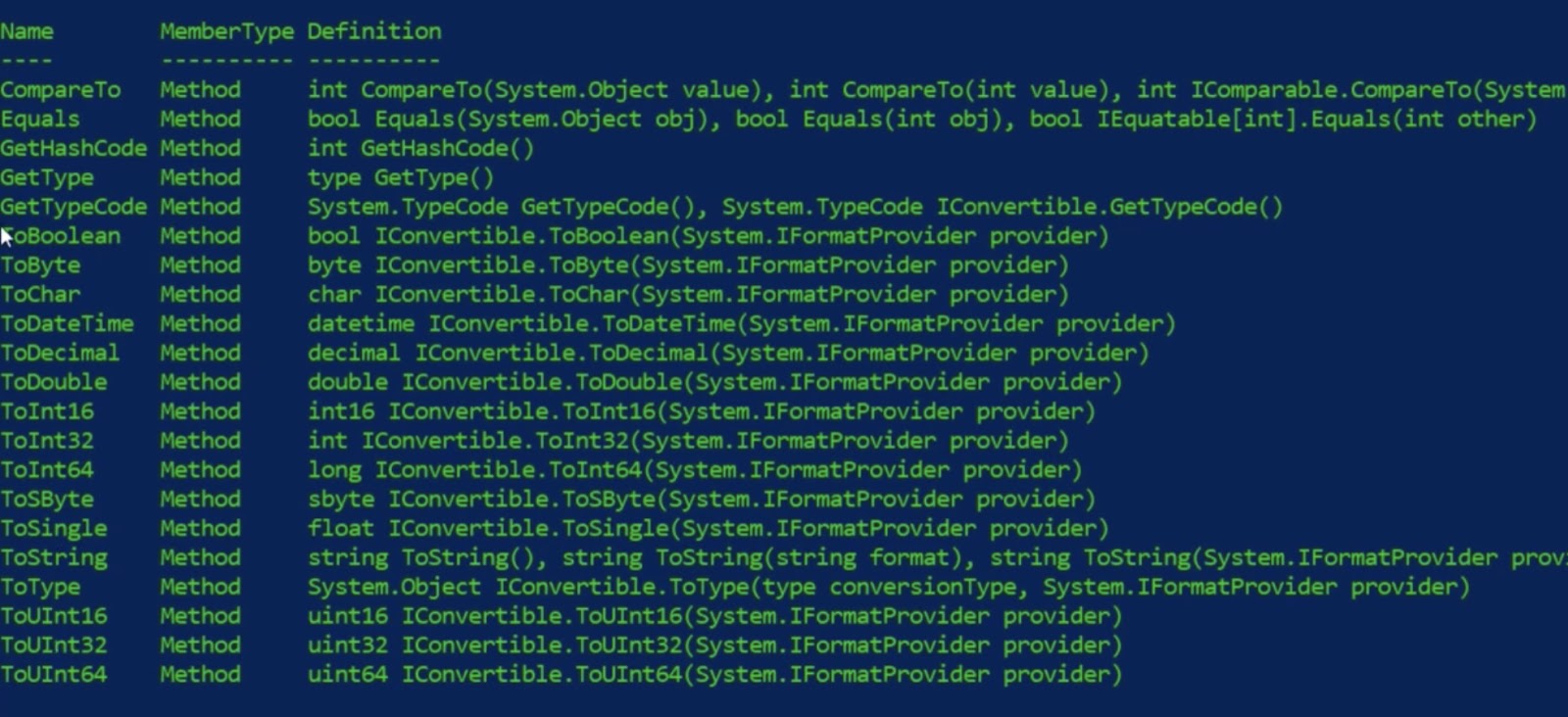
Mastering ‘Set-Variable’ in PowerShell: Efficient Scripting
PowerShell, as a versatile scripting language, offers support for constants and immutable variables. These read-only variables, impervious to standard alteration, are established through the deployment of the ‘New-Variable’ command with the ‘-Option ReadOnly’ parameter.
Creating a Read-Only Variable
To initiate a read-only variable, one can employ the following command:
```powershell
New-Variable -Name myVar -Value 1337 -Option ReadOnly
```
Accessing the Read-Only Variable
Accessing the value of this variable is straightforward:
```powershell
$myVar
```
Attempting Modification
However, any attempt to modify this variable in the conventional manner would yield an error:
```powershell
$myVar = 31337
```
The following error message will be encountered:
```powershell
Cannot overwrite variable myVar because it is read-only or constant.
At line:1 char:1
+ $myVar = 31337
+ ~~~~~~~~~~~~~~
+ CategoryInfo : WriteError: (myVar:String) [], SessionStateUnauthorizedAccessException
+ FullyQualifiedErrorId : VariableNotWritable
```
Overcoming Read-Only Status
To alter a read-only variable, the ‘-Force’ parameter must be employed. Here’s how you can do it:
```powershell
$myvar # Should output 1337
New-Variable -Name myVar -Value 31337 -Option ReadOnly -Force
```
After applying this command, the value of ‘myVar’ is indeed altered:
```powershell
$myVar # Should output 31337
```
In this particular scenario, the ‘-Force’ parameter allows for the modification of the read-only variable’s value.